The NSURL class is a subclass of the NSObject class and it provides methods for creating and manipulating URL addresses. URL stands for “Universal Resource Locator”. Here are few things you should know about the NSURL class:
- It is the only class spelt with all upper case.
- an NSURL object is not a string object such as @"http:/www.theapplady.net"
- There are many methods in the Foundation Framework that take an NSURL object as an argument.
To practice source code presented in this lesson, you should download and unzip this file.
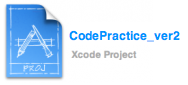
Launch the project (CodePractice.xcodeproj) and enter code in the viewController.m file’s buttonTapped method, then click the “Click Me” button to execute the method’s code. |
Common NSURL Methods
The NSURL class contain several methods for manipulating NSURL objects. In this section I show how to use three of them.
The URLWithString: Method
This method creates and returns an NSUL object. The argument passed to the method must be an NSString which is a valid website address.
Code |
Output |
// Instantiate and initialize an NSURL object
NSURL *websiteAddress = [NSURL URLWithString:@"http://www.theapplady.net"];
// Place the websistAddress's host name and URL in separate NSString objects
NSString *host = [websiteAddress host];
NSString *url = [websiteAddress absoluteString];
// Display the websistAddress's host name and URL in the UITextView control
self.outputBox.text = [NSString stringWithFormat:@"HOST:\n%@\n\nURL:\n%@",host,url];
|
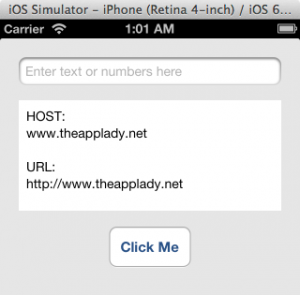 |
The fileURLWithPath: Method
This method is used for creating and returning an NSURL object as a file URL. The required argument passed to the method is a valid path to a file.
Code |
Output |
// Instantiate and initialize an NSURL object
NSURL *textFile = [NSURL fileURLWithPath:@"/Users/YOUR_NAME/Downloads/testfile.txt"];
// Place the file's host name and URL in separate NSString objects
NSString *host = [textFile host];
NSString *url = [textFile absoluteString];
// Display the file's host name and URL in the UITextView control
self.outputBox.text = [NSString stringWithFormat:@"HOST:\n%@\n\nURL:\n%@",host,url];
|
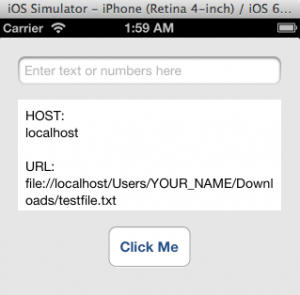 |
The requestWithURL: Method
This method creates and returns an URL request object, which you can display in a UIWebView control, as demonstrated below.
Code |
Output |
// Instantiate and initialize an NSURL object
NSURL *websiteAddress = [NSURL URLWithString:@"https://mail.google.com"];
// Create an NSURLRequst object
NSURLRequest *webPage = [NSURLRequest requestWithURL:websiteAddress];
// Load webpage in the UIWebView control
[self.webView loadRequest:webPage];
|
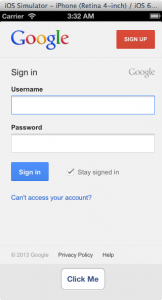 |
No Responses