You’ve already learned how to use methods to instantiate and initialize instances of these classes: NSNumber, NSArray, NSDictionary. Now, I will show you how to use Objective-C literals to initialize instances of above mentioned classes. By the way, the NSArray and NSDictionary are known as collection classes.
NSNumber Literal
The NSNumber literal provide a syntax for boxing C literal so you can add it in a (NSSet, NSArray, NSDictionary) collection object. To box a C int, float, or double literal, put an @ before the number, such as @295, and the compiler implicitly wraps that number in the appropriate box type.
Example
// Box C numbers
NSNumber *anInteger = @44;
NSNumber *afloat = @23.17f;
NSNumber *aDouble = @12890.78;
// Method #1: Create and initialize a collection with three boxed numbers
NSArray *numbers = @[anInteger,afloat,aDouble];
// Method #2: Create and initialize a collection with three boxed numbers
NSArray *numbers = [NSArray arrayWithObjects:@44, @23.17f, @12890.78, nil];
It is possible to box a C expression using the @() syntax, then place the expression result into an NSNumber object.
Example Code
// Box two C numbers
double productPrice = 50.75;
double taxRate = .15;
// Box arithmetic expression, then place the result in an NSNumber object
NSNumber *taxAmount = @(productPrice * taxRate);
NSLog(@"%.2f", [taxAmount doubleValue]);
NSArray Literal
The NSArray literal provides a shorthand for initializing an NSArray object.
Example Code
|
Output
|
// Create an array of objects using the literal syntax to initialize the array
NSArray *browserIds = [NSArray arrayWithObjects:@01, @02, @03, @04, nil];
// Create an array and initialize it with the browserIds array and string literals
NSArray *browserIdsAndNames = @[browserIds, @"Safari", @"ThunderBird", @"Chrome", @"Opera"];
// Convert the immutable NSArray to a mutable array
NSMutableArray *webBrowsers = [browserIdsAndNames mutableCopy];
// Convert the first element of the webBrowsers array before assigning it to a string object
NSString *stringIds = [[webBrowsers objectAtIndex:0] componentsJoinedByString:@"\n"];
// Remove the first element from the mutable array
[webBrowsers removeObjectAtIndex:0];
// Convert the webBrowsers elements to a string
NSString *stringNames = [webBrowsers componentsJoinedByString:@"\n"];
// Concatenate strings
NSString *finalOutput = [stringIds stringByAppendingFormat:@"\n%@", stringNames];
// Display concatenated strings
self.outputBox.text = finalOutput;
|
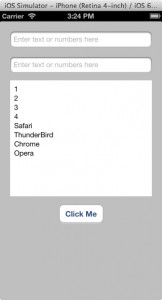 |
NSDictionary Literal
The NS literal provides a shorthand for initializing an NSDictionary object.
Example Code
|
Output
|
// Create an dictionary of objects using the literal syntax {key:value} to initialize the dictionary
NSDictionary *browserObjects = @{@"id01":@"Safari",
@"id02":@"ThunderBird",
@"id03":@"Chrome",
@"id04":@"Opera"};
// Place the dictionary's keys and values in separate arrays
NSArray *dicKeys = [browserObjects allKeys];
NSArray *dicValues = [browserObjects allValues];
// Convert them to string objects
NSString *stringDicKeys = [dicKeys componentsJoinedByString:@"\n"];
NSString *stringDicValues = [dicValues componentsJoinedByString:@"\n"];
// Display the dictionary keys and values
self.outputBox.text = [stringDicKeys stringByAppendingFormat:@"\n---------------\n%@",stringDicValues];
// Two ways of displaying a value of the dictionary object in a text field control
self.inputBoxOne.text = [browserObjects objectForKey:@"id03"];
NSString *object = browserObjects[@"id01"];
self.inputBoxTwo.text = object;
|
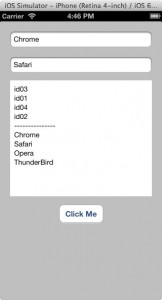 |
Literal Limitation
The Objective-C language does not supporting NSSet, NSMutableArray, or NSMutableDictionary literal. In other words, you cannot initialize instances of these objects using the literal syntax.